Embedded checkout form
For maximum styling and customizability, you can embed our payment form on your website.
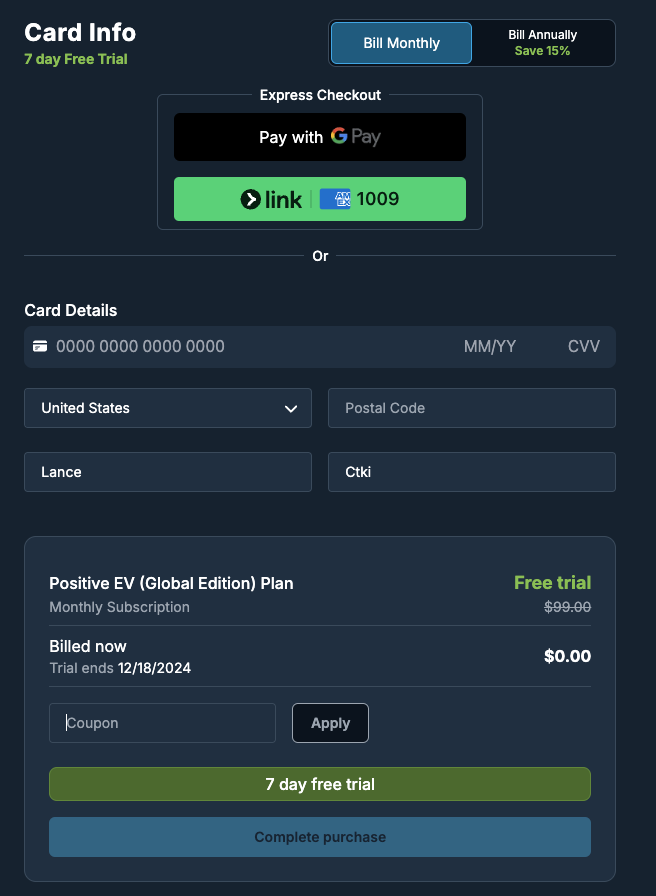
Once a customer has submitted the checkout form, this will automatically sync into our system and you will see the customer in your OpenPay admin console with the subscription or one-time price that they purchased.
Install and import our clientCopied!
For this recipe, we will use the Python SDK, which you can install using pip install getopenpay
or poetry add getopenpay
from getopenpay.client import ApiKeys, OpenPayClient
OP_PUBLISHABLE_TOKEN = 'TODO_YOUR_PUBLISHABLE_TOKEN'
OP_SECRET_TOKEN = 'TODO_YOUR_SECRET_TOKEN'
api_keys = ApiKeys(publishable_key=OP_PUBLISHABLE_TOKEN, secret_key=OP_SECRET_TOKEN)
# sandbox/staging environment
sandbox_host = 'https://connto.openpaystaging.com'
# production environment
production_host = 'https://connto.getopenpay.com'
client = OpenPayClient(api_keys=api_keys, host=sandbox_host)
Create a secure checkout tokenCopied!
You'll need to create a Checkout Session with mode: 'SUBSCRIPTION'. This will result in checkout session information that contains a secure_token to be used in the next steps
Once the secure_token is generated, it’s ready to be used on the frontend to securely collect payment details.
response = self.client.checkout.create_checkout_session(
CreateCheckoutSessionRequest(
customer_id='TODO_TARGET_CUSTOMER_ID', # (Optional)
customer_email=None,
line_items=[
CreateCheckoutLineItem(price_id='TODO_DESIRED_PRICE_1', quantity=2),
CreateCheckoutLineItem(price_id='TODO_DESIRED_PRICE_2', quantity=1),
],
currency=CurrencyEnum.USD,
mode=CheckoutMode.SUBSCRIPTION,
return_url=RETURN_URL,
success_url=SUCCESS_URL,
)
)
# token to be passed to frontend
print(f'Secure token: {response.secure_token}')
Implement the payment formCopied!
Use the component to display a secure form where customers can enter their payment details. You will need to render OpenPay Card Elements as well as required billing information to proceed checkout.
### Embed the form on your UI
import {
ElementsForm,
FieldName,
CardElement,
} from '@getopenpay/openpay-js-react';
import { FC, PropsWithChildren, useState } from 'react';
interface InputProps {
type?: string;
placeholder: string;
openPayId?: FieldName;
}
const Input: FC<InputProps> = ({ type, placeholder, openPayId }) => (
<input
type={type ?? 'text'}
placeholder={placeholder}
className="w-full text-sm bg-transparent outline-none py-2"
data-opid={openPayId}
/>
);
const StyledWrapper: FC<PropsWithChildren> = (props) => {
const { children } = props;
return <div className="bg-emerald-50 dark:bg-emerald-800 shadow-md px-4 py-2 rounded-md mb-2">{children}</div>;
};
const HorizontalRule: FC = () => <hr className="border-emerald-200 dark:border-emerald-700" />;
function CheckoutForm() {
const [error, setError] = useState<string | undefined>(undefined);
const [amount, setAmount] = useState<string | undefined>(undefined);
const onLoad = (totalAmountAtoms: number) => {
setAmount(`$${totalAmountAtoms / 100}`);
};
const onCheckoutError = (message: string) => {
setError(message);
};
const onCheckoutSuccess = (invoiceUrls: string[], subscriptionIds: string[], customerId: string) => {
console.log('invoiceUrls', invoiceUrls); // Can be used to redirect or display invoices
console.log('subscriptionIds', subscriptionIds); // Useful for retriving details about the subscription
console.log('customerId', customerId) // Created customer's ID or pre-assigned customer's ID
};
return (
<ElementsForm
baseUrl={isStagingEnv ? "https://cde.openpaystaging.com": undefined}
checkoutSecureToken={`checkout-secure-token-uuid`}
onChange={() => setError(undefined)}
onLoad={onLoad}
onValidationError={(message) => setError(message)}
onCheckoutSuccess={onCheckoutSuccess}
onCheckoutError={onCheckoutError}
>
{({ submit }) => (
<>
<StyledWrapper>
<div className="flex gap-2 items-center justify-between">
<Input placeholder="Given name" openPayId={FieldName.FIRST_NAME} />
<Input placeholder="Family name" openPayId={FieldName.LAST_NAME} />
</div>
<HorizontalRule />
<Input placeholder="Email" type="email" openPayId={FieldName.EMAIL} />
<HorizontalRule />
<div className="flex gap-2 items-center justify-between">
<Input placeholder="Country" openPayId={FieldName.COUNTRY} />
<Input placeholder="ZIP" openPayId={FieldName.ZIP_CODE} />
</div>
</StyledWrapper>
<StyledWrapper>
<CardElement />
</StyledWrapper>
{error && <small className="font-bold text-xs mt-2 text-red-500">{error}</small>}
<button
onClick={submit}
className="px-4 py-2 mt-2 w-full font-bold rounded-lg bg-emerald-500 text-white hover:bg-emerald-400 active:bg-emerald-600"
>
Pay {amount}
</button>
</>
)}
</ElementsForm>
);
}
Key callbacks and props:
-
checkoutSecureToken
: The secure token generated from the backend to link the session. -
onLoad
: Triggered when the checkout form is successfully loaded. It includestotalAmountAtoms
andcurrency
. -
onValidationError
: Handles any validation errors from the form fields. -
onCheckoutSuccess
: A callback that returns theinvoiceUrls
,subscriptionIds
andcustomerId
after the checkout process is successful. -
onCheckoutError
: Handles any checkout error during the process.
For detailed props and callbacks, please refer to the main guide:
Guide (React)Integrating payment elements
Handling a successful checkoutCopied!
Once the customer successfully checkout with their payment method, the onCheckoutSuccess
callback is triggered. The callback returns the invoiceUrls
, subscriptionIds
and customerId
.
-
Invoice urls can be used to display invoices or redirect to invoice page
-
Subscription IDs can be used for further processing with OpenPay SDK
-
Customer ID is the created customer on OpenPay side during checkout process. If you are creating the checkout session for specific customer, it will return that specific customer ID
Install and import our clientCopied!
For this recipe, we will use the Python SDK, which you can install using pip install getopenpay
or poetry add getopenpay
from getopenpay.client import ApiKeys, OpenPayClient
OP_PUBLISHABLE_TOKEN = 'TODO_YOUR_PUBLISHABLE_TOKEN'
OP_SECRET_TOKEN = 'TODO_YOUR_SECRET_TOKEN'
api_keys = ApiKeys(publishable_key=OP_PUBLISHABLE_TOKEN, secret_key=OP_SECRET_TOKEN)
# sandbox/staging environment
sandbox_host = 'https://connto.openpaystaging.com'
# production environment
production_host = 'https://connto.getopenpay.com'
client = OpenPayClient(api_keys=api_keys, host=sandbox_host)
Create a secure checkout tokenCopied!
You'll need to create a Checkout Session with mode: 'SUBSCRIPTION'. This will result in checkout session information that contains a secure_token to be used in the next steps
Once the secure_token is generated, it’s ready to be used on the frontend to securely collect payment details.
response = self.client.checkout.create_checkout_session(
CreateCheckoutSessionRequest(
customer_id='TODO_TARGET_CUSTOMER_ID', # (Optional)
customer_email=None,
line_items=[
CreateCheckoutLineItem(price_id='TODO_DESIRED_PRICE_1', quantity=2),
CreateCheckoutLineItem(price_id='TODO_DESIRED_PRICE_2', quantity=1),
],
currency=CurrencyEnum.USD,
mode=CheckoutMode.SUBSCRIPTION,
return_url=RETURN_URL,
success_url=SUCCESS_URL,
)
)
# token to be passed to frontend
print(f'Secure token: {response.secure_token}')
Implement the payment form in pureCopied!
Use the component to display a secure form where customers can enter their payment details. You will need to render OpenPay Card Elements as well as required billing information to proceed checkout.
### Embed the form on your UI
import {
ElementsForm,
FieldName,
CardElement,
} from '@getopenpay/openpay-js-react';
import { FC, PropsWithChildren, useState } from 'react';
interface InputProps {
type?: string;
placeholder: string;
openPayId?: FieldName;
}
const Input: FC<InputProps> = ({ type, placeholder, openPayId }) => (
<input
type={type ?? 'text'}
placeholder={placeholder}
className="w-full text-sm bg-transparent outline-none py-2"
data-opid={openPayId}
/>
);
const StyledWrapper: FC<PropsWithChildren> = (props) => {
const { children } = props;
return <div className="bg-emerald-50 dark:bg-emerald-800 shadow-md px-4 py-2 rounded-md mb-2">{children}</div>;
};
const HorizontalRule: FC = () => <hr className="border-emerald-200 dark:border-emerald-700" />;
function CheckoutForm() {
const [error, setError] = useState<string | undefined>(undefined);
const [amount, setAmount] = useState<string | undefined>(undefined);
const onLoad = (totalAmountAtoms: number) => {
setAmount(`$${totalAmountAtoms / 100}`);
};
const onCheckoutError = (message: string) => {
setError(message);
};
const onCheckoutSuccess = (invoiceUrls: string[], subscriptionIds: string[], customerId: string) => {
console.log('invoiceUrls', invoiceUrls); // Can be used to redirect or display invoices
console.log('subscriptionIds', subscriptionIds); // Useful for retriving details about the subscription
console.log('customerId', customerId) // Created customer's ID or pre-assigned customer's ID
};
return (
<ElementsForm
baseUrl={isStagingEnv ? "https://cde.openpaystaging.com": undefined}
checkoutSecureToken={`checkout-secure-token-uuid`}
onChange={() => setError(undefined)}
onLoad={onLoad}
onValidationError={(message) => setError(message)}
onCheckoutSuccess={onCheckoutSuccess}
onCheckoutError={onCheckoutError}
>
{({ submit }) => (
<>
<StyledWrapper>
<div className="flex gap-2 items-center justify-between">
<Input placeholder="Given name" openPayId={FieldName.FIRST_NAME} />
<Input placeholder="Family name" openPayId={FieldName.LAST_NAME} />
</div>
<HorizontalRule />
<Input placeholder="Email" type="email" openPayId={FieldName.EMAIL} />
<HorizontalRule />
<div className="flex gap-2 items-center justify-between">
<Input placeholder="Country" openPayId={FieldName.COUNTRY} />
<Input placeholder="ZIP" openPayId={FieldName.ZIP_CODE} />
</div>
</StyledWrapper>
<StyledWrapper>
<CardElement />
</StyledWrapper>
{error && <small className="font-bold text-xs mt-2 text-red-500">{error}</small>}
<button
onClick={submit}
className="px-4 py-2 mt-2 w-full font-bold rounded-lg bg-emerald-500 text-white hover:bg-emerald-400 active:bg-emerald-600"
>
Pay {amount}
</button>
</>
)}
</ElementsForm>
);
}
Key callbacks and props:
-
checkoutSecureToken
: The secure token generated from the backend to link the session. -
onLoad
: Triggered when the checkout form is successfully loaded. It includestotalAmountAtoms
andcurrency
. -
onValidationError
: Handles any validation errors from the form fields. -
onCheckoutSuccess
: A callback that returns theinvoiceUrls
,subscriptionIds
andcustomerId
after the checkout process is successful. -
onCheckoutError
: Handles any checkout error during the process.
For detailed props and callbacks, please refer to the main guide:
Guide (.js)Integrating payment elements
Handling a successful checkoutCopied!
Once the customer successfully checkout with their payment method, the onCheckoutSuccess
callback is triggered. The callback returns the invoiceUrls
, subscriptionIds
and customerId
.
-
Invoice urls can be used to display invoices or redirect to invoice page
-
Subscription IDs can be used for further processing with OpenPay SDK
-
Customer ID is the created customer on OpenPay side during checkout process. If you are creating the checkout session for specific customer, it will return that specific customer ID